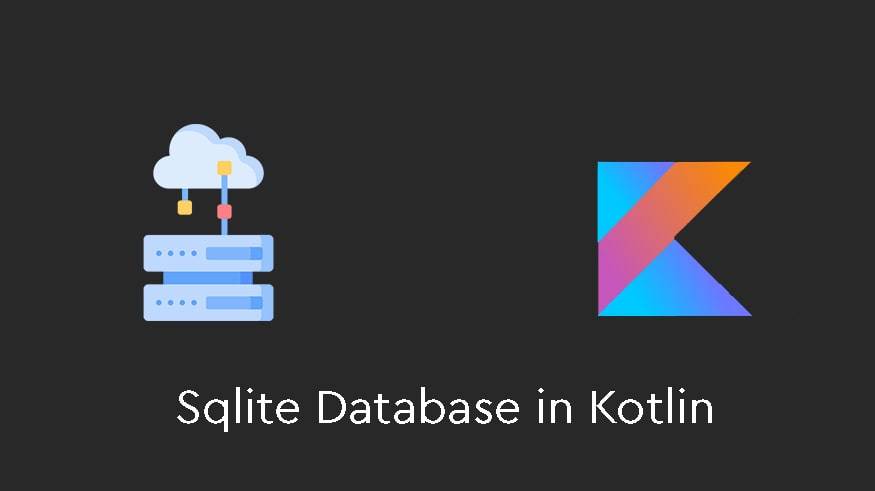
We will teach the SQLite database in Kotlin. The sqlite database is one of the most famous databases in Android. It is used to store data. Using the sqlite database in Kotlin is very similar to Java. Follow us to learn how to use the sqlite database. take
You don’t need to add a special library to use the Sqlite database.
The first step to use the sqlite database is to create a DatabaseHandler or DatabaseHelper. In fact, by using these types of classes, the operations of inserting and updating will be easier to read.
Enter your layer called activity_main and put the following codes.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical"> <EditText android:id="@+id/editTextName" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="10dp" android:padding="8dp" /> <EditText android:id="@+id/editTextAge" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="10dp" android:autofillHints="Age" android:inputType="number" android:padding="8dp" android:textColor="@android:color/background_dark" /> <Button android:id="@+id/btnInsert" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="10dp" android:padding="8dp" android:text="Add data" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:orientation="horizontal" android:weightSum="3"> <Button android:id="@+id/btnRead" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_margin="10dp" android:layout_weight="1" android:padding="8dp" android:text="Read" /> </LinearLayout> <ScrollView android:layout_width="match_parent" android:layout_height="match_parent> <TextView android:id="@+id/tvResult" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="8dp" android:textSize="16sp" android:textStyle="bold" /> </ScrollView> </LinearLayout>
Above we have two input fields that enter the user’s information in the SQlite database and a button that shows the information entered in the database.
Create a class called DataBaseHelper.kt and put the following code in it.
import android.content.ContentValues import android.content.Context import android.database.sqlite.SQLiteDatabase import android.database.sqlite.SQLiteOpenHelper import android.widget.Toast val DATABASENAME = "MY DATABASE" val TABLENAME = "Users" val COL_NAME = "name" val COL_AGE = "age" val COL_ID = "id" class DataBaseHandler(var context: Context) : SQLiteOpenHelper(context, DATABASENAME, null, 1) { override fun onCreate(db: SQLiteDatabase?) { val createTable = "CREATE TABLE " + TABLENAME + " (" + COL_ID + " INTEGER PRIMARY KEY AUTOINCREMENT," + COL_NAME + " VARCHAR(256)," + COL_AGE + " INTEGER)" db?.execSQL(createTable) } override fun onUpgrade(db: SQLiteDatabase?, oldVersion: Int, newVersion: Int) { //onCreate(db); } fun insertData(user: User) { val database = this.writableDatabase val contentValues = ContentValues() contentValues.put(COL_NAME, user.name) contentValues.put(COL_AGE, user.age) val result = database.insert(TABLENAME, null, contentValues) if (result == (0).toLong()) { Toast.makeText(context, "Failed", Toast.LENGTH_SHORT).show() } else { Toast.makeText(context, "Success", Toast.LENGTH_SHORT).show() } } fun readData(): MutableList<User> { val list: MutableList<User> = ArrayList() val db = this.readableDatabase val query = "Select * from $TABLENAME" val result = db.rawQuery(query, null) if (result.moveToFirst()) { do { val user = User() user.id = result.getString(result.getColumnIndex(COL_ID)).toInt() user.name = result.getString(result.getColumnIndex(COL_NAME)) user.age = result.getString(result.getColumnIndex(COL_AGE)).toInt() list.add(user) } while (result.moveToNext()) } return list } }
onCreate creates the database.
onUpgrade: If the database is updated, the database will be dropped once and then created from scratch.
insertData: Insertion is done by this method.
readData: shows the information stored in the database.
To use this class, we enter MainActivity.kt and call the subclass.
import androidx.appcompat.app.AppCompatActivity import android.os.Bundle import android.widget.Toast import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) title = "KotlinApp" val context = this val db = DataBaseHandler(context) btnInsert.setOnClickListener { if (editTextName.text.toString().isNotEmpty() && editTextAge.text.toString().isNotEmpty() ) { val user = User(editTextName.text.toString(), editTextAge.text.toString().toInt()) db.insertData(user) clearField() } else { Toast.makeText(context, "Please Fill All Data's", Toast.LENGTH_SHORT).show() } } btnRead.setOnClickListener { val data = db.readData() tvResult.text = "" for (i in 0 until data.size) { tvResult.append( data[i].id.toString() + " " + data[i].name + " " + data[i].age + "\n" ) } } } private fun clearField() { editTextName.text.clear() editTextAge.text.clear() } }
The above code receives the information from EditText and inserts the received information into the database, and it is also possible to see the stored data.
be successful and victorious.